One question I often get asked is how one could create an animated splash screen for their Ionic applications – the bad news is it’s not possible to animate a splash screen (and that’s not just an Ionic thing, it’s not possible in general). A splash screen is a static PNG image with no transparency.
Although it’s not possible to animate the splash screen itself, we can still create an effect so that it looks like the splash screen is animated. We can do this by having our application displays its own “fake” splash screen after the real splash screen is displayed (but we can hide the transition from the fake splash screen to the real splash screen so that, to the user, it would just look like the splash screen is animated).
The basic idea goes like this:
- Generate a splash screen that can transition seamlessly to your fake splash screen (e.g. just a solid colour)
- Set the splash screen so that it does not auto hide
- Once the application has loaded, launch your fake splash screen page that contains the animation
- Hide the real splash screen
- After a set amount of time, dismiss the fake splash screen
This is what it will look like when it is completed:
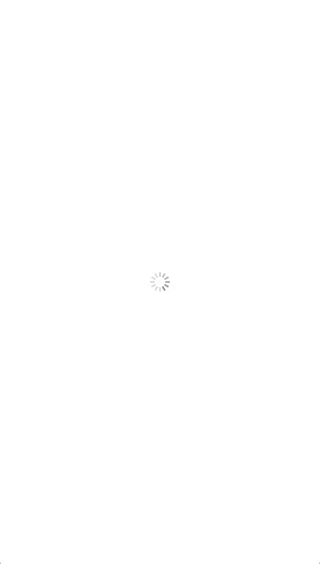
I am going to be using the SVG animation that I built in this tutorial, so if you would like to see how to create the animation itself you should read that tutorial.
Before We Get Started
Last updated for Ionic 3.9.2
Before you go through this tutorial, you should have at least a basic understanding of Ionic concepts. You must also already have Ionic set up on your machine.
If you’re not familiar with Ionic already, I’d recommend reading my Ionic Beginners Guide or watching my beginners series first to get up and running and understand the basic concepts. If you want a much more detailed guide for learning Ionic, then take a look at Building Mobile Apps with Ionic.
1. Generate a New Ionic Application
We’re going to start by generating a new blank Ionic application, to do that just run the following command:
ionic start ionic-animated-splashscreen blank
Once that has finished generating, you should make it your working directory:
cd ionic-animated-splashscreen
We will be generating a new page to serve as our fake splash screen, so let’s do that now:
ionic g page Splash
We will also need to make sure to set that splash screen up in our app.module.ts file.
Modify src/app/app.module.ts to reflect the following:
import { BrowserModule } from '@angular/platform-browser';
import { ErrorHandler, NgModule } from '@angular/core';
import { IonicApp, IonicErrorHandler, IonicModule } from 'ionic-angular';
import { SplashScreen } from '@ionic-native/splash-screen';
import { StatusBar } from '@ionic-native/status-bar';
import { MyApp } from './app.component';
import { HomePage } from '../pages/home/home';
import { Splash } from '../pages/splash/splash';
@NgModule({
declarations: [MyApp, HomePage, Splash],
imports: [BrowserModule, IonicModule.forRoot(MyApp)],
bootstrap: [IonicApp],
entryComponents: [MyApp, HomePage, Splash],
providers: [
StatusBar,
SplashScreen,
{ provide: ErrorHandler, useClass: IonicErrorHandler },
],
})
export class AppModule {}
2. Create the Real Splash Screen
This is the easy part – all we need to do is create a simple splash screen and configure so that it never hides automatically. The animation we want to display on our fake splash screen will look like this:
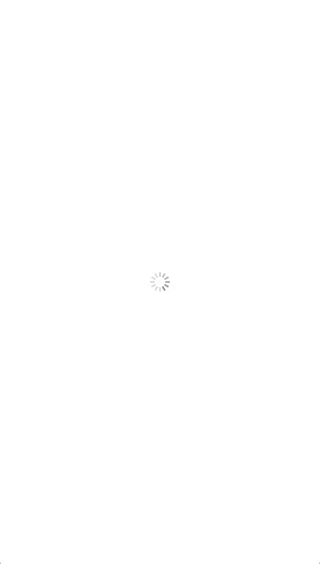
It’s just the logo sitting on a white background. So that the user won’t notice the transition from the real splash screen to the fake one, we should make the real splash screen just a completely white blank image (i.e. it will literally have nothing on it, not even the logo, it should just be completely white).
If you need help with generating splash screens, take a look at this: Automatically Generate Splash Screens and Icons with Ionic CLI.
In order to make sure that this splash screen does not get hidden automatically, you will need to include the following configurations in your config.xml file:
<preference name="FadeSplashScreen" value="false" />
<preference name="AutoHideSplashScreen" value="false" />
and you will need to remove the automatically generated call to hide the splash screen in src/app/app.component.ts:
constructor(platform: Platform, statusBar: StatusBar, splashScreen: SplashScreen) {
platform.ready().then(() => {
statusBar.styleDefault();
// splashScreen.hide(); // REMOVE THIS!
});
}
3. Create the Fake Splash Screen
We have our real splash screen sorted, so now we just need to implement our fake splash screen and have that hide the real one after it has launched. Let’s first modify the template to display the SVG animation.
Modify src/pages/splash/splash.html to reflect the following:
<ion-content>
<svg id="bars" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 63.15 224.35">
<defs>
<style>
.cls-1 {
fill: #dd238c;
}
.cls-2 {
fill: #ef4328;
}
.cls-3 {
fill: #7dd0df;
}
.cls-4 {
fill: #febf12;
}
.cls-5 {
fill: #282828;
}
</style>
</defs>
<title>jmlogo</title>
<rect class="cls-1" x="27.22" width="20.06" height="163.78" />
<rect class="cls-2" y="4" width="20.06" height="163.78" />
<rect class="cls-3" x="13.9" y="13.1" width="20.06" height="163.78" />
<rect class="cls-4" x="43.1" y="7.45" width="20.06" height="163.78" />
<path
class="cls-5"
d="M243.5,323a12,12,0,0,1-.5,3.43,8.88,8.88,0,0,1-1.63,3.1,8.24,8.24,0,0,1-3,2.26,10.8,10.8,0,0,1-4.58.86,9.63,9.63,0,0,1-6-1.82,8.48,8.48,0,0,1-3.07-5.47l4-.82a5.64,5.64,0,0,0,1.66,3.19,4.86,4.86,0,0,0,3.43,1.18,5.71,5.71,0,0,0,2.83-.62,4.53,4.53,0,0,0,1.7-1.63,7,7,0,0,0,.84-2.33,15.15,15.15,0,0,0,.24-2.71V297.82h4V323Z"
transform="translate(-224.04 -108.31)"
/>
<path
class="cls-5"
d="M252,297.82h6l11.52,26.64h0.1l11.62-26.64H287v34h-4V303.29h-0.1L270.72,331.8h-2.45l-12.19-28.51H256V331.8h-4v-34Z"
transform="translate(-224.04 -108.31)"
/>
</svg>
</ion-content>
Modify src/pages/splash/splash.scss to reflect the following:
.ios,
.md {
page-splash {
.scroll-content {
display: flex;
justify-content: center;
align-items: center;
}
#bars {
height: 80%;
.cls-1 {
opacity: 0;
transform: rotate3d(1, 1, 0, 50deg);
animation: 1s 0.2s ease fadeInAndSpin;
animation-fill-mode: forwards;
}
.cls-2 {
opacity: 0;
transform: rotate3d(1, 1, 0, 50deg);
animation: 1s 0.4s ease fadeInAndSpin;
animation-fill-mode: forwards;
}
.cls-3 {
opacity: 0;
transform: rotate3d(1, 1, 0, 50deg);
animation: 1s 0.6s ease fadeInAndSpin;
animation-fill-mode: forwards;
}
.cls-4 {
opacity: 0;
transform: rotate3d(1, 1, 0, 50deg);
animation: 1s 0.8s ease fadeInAndSpin;
animation-fill-mode: forwards;
}
.cls-5,
.cls-6 {
opacity: 0;
animation: 2s 1.5s linear fadeIn;
animation-fill-mode: forwards;
}
}
}
}
@keyframes fadeInAndSpin {
100% {
opacity: 1;
transform: rotate3d(1, 1, 0, 0deg);
}
}
@keyframes fadeIn {
100% {
opacity: 1;
}
}
We will also need to have that modal automatically launch itself when the application starts.
Modify src/app/app.component.ts to reflect the following:
import { Component } from '@angular/core';
import { Platform, ModalController } from 'ionic-angular';
import { StatusBar } from '@ionic-native/status-bar';
import { SplashScreen } from '@ionic-native/splash-screen';
import { HomePage } from '../pages/home/home';
import { Splash } from '../pages/splash/splash';
@Component({
templateUrl: 'app.html',
})
export class MyApp {
rootPage: any = HomePage;
constructor(
platform: Platform,
statusBar: StatusBar,
splashScreen: SplashScreen,
modalCtrl: ModalController
) {
platform.ready().then(() => {
statusBar.styleDefault();
let splash = modalCtrl.create(Splash);
splash.present();
});
}
}
As soon as the application is ready, we create a modal using the splash page and have it launch automatically. Now we need to make sure that the modal handles hiding the real splash screen, and also hides itself once the animation is complete.
Modify src/pages/splash/splash.ts to reflect the following:
import { Component } from '@angular/core';
import { ViewController } from 'ionic-angular';
import { SplashScreen } from '@ionic-native/splash-screen';
@Component({
selector: 'page-splash',
templateUrl: 'splash.html',
})
export class Splash {
constructor(public viewCtrl: ViewController, public splashScreen: SplashScreen) {
}
ionViewDidEnter() {
this.splashScreen.hide();
setTimeout(() => {
this.viewCtrl.dismiss();
}, 4000);
}
}
We hook into ionViewDidEnter
which will run as soon as the modal launches. In here, we hide the splash screen and then after four seconds we also dismiss this modal. Once this modal is dismissed the user will be in the main application.
On smaller devices, a modal will take up the full width and height of a screen by default, so it works well for our fake splash screen. On larger devices like a tablet, the modal does not take up the entire screen by default, so we will need to fix that.
Modify src/app/app.scss to include the following style:
.modal-wrapper {
width: 100%;
height: 100%;
top: 0;
left: 0;
}
If you attempt to launch your application on a device now, you should see something like this:
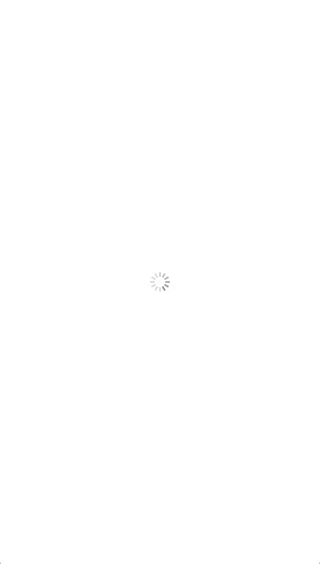
Summary
Even though it’s technically impossible to create an actual splash screen that is animated, we have pretty much achieved the same thing here. Really, the user is viewing two different splash screens, but the end result is that it just looks like a single animated splash screen.