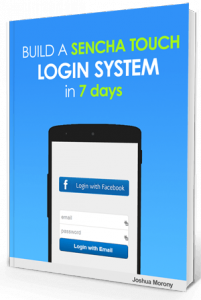
Hey there! There is also a (very) expanded version of this tutorial series available that includes the Facebook login portion. As well as getting a convenient PDF, ePub and mobi version, you'll also get a premium video tutorial series covering the development from start to finish and a bunch of other goodies! Check it out here .
Welcome to Part 1 of my tutorial series where I walk you through how to create a Sencha Touch application with a email + facebook signup and authentication system. If you haven’t seen it yet, make sure to start at Part 1. Here’s the rest of the series:
Part 1: Setting up the Database and API
Part 2: Creating the Application Screens
Part 3: Creating the User Model and API
Part 4: Finishing off the Logic
One really common pattern found in mobile applications is a dual email + Facebook log in system. Facebook is a very popular sign in method, but not everybody has an account and not everybody wants to use their Facebook account to sign in to new services. This makes giving the option to users to use either email or their Facebook account to sign up very attractive.
There seems to be very little (that I could find at least) information out there on how to set up a log in / authentication system in Sencha Touch. So I’m writing a series of blog posts that will cover how to set up a log in system from scratch with the option to sign up with either email or a Facebook account. The application will have a PHP & MySQL backend and will also use PhoneGap Build.
Topics covered over the coming weeks will include:
- Setting up the database and API (this post)
- Creating the application and screens
- Email sign up system
- Authentication and generating sessions for users
- Auto login / remember me for return visits
- Integrating a Facebook signup system
In this first part, I will walk you through setting up your database and setting up some PHP files on your server that are ready to receive calls from your application.
Setting up the database
We’re going to have to create a MySQL database first of course so go ahead and do that, calling it whatever you wish. Once you’ve created the database, add the following table to it:
CREATE TABLE `users` (
`id` INT(11) UNSIGNED NOT NULL AUTO_INCREMENT,
`fbid` VARCHAR(255),
`email` VARCHAR(100) NOT NULL,
`password` VARCHAR(255) NOT NULL,
`session` VARCHAR(255),
PRIMARY KEY (`id`)
);
This will allow us to store the details the users uses to sign up, as well as any other details we would like to track. You could go ahead and add some other fields like ‘first_name’, ‘phone’ and so on if you wish. If you’re building out a real application, you’re likely going to require other tables as well, but this is all we need to actually get the log in system working.
Setting up the API
Throughout the log in and sign up processes, our application will be making calls to an API hosted on the Internet. This API will be created with PHP files which will interact with our database, and then return data in a JSON format to our application.
Although we are not implementing it just yet, our application will make calls to our server using an Ajax proxy. The problem with this is that we can run into some Cross Origin Resource Sharing errors which might look something like the following:
XMLHttpRequest cannot load [URL]. No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost' is therefore not allowed access. XMLHttpRequest cannot load [URL]. Origin [URL] is not allowed by Access-Control-Allow-Origin. Request header field Content-Type is not allowed by Access-Control-Allow-HeadersEssentially, we’re running into security problems because we’re making requests to a domain different to the one the application is hosted on. I wrote a blog posts on this recently so if you’d like more information you can check it out here.
What we want to do now though is make sure we don’t run into any of these errors by making sure we set our headers (and everything else) correctly from the beginning. Now I need you to create a file called ‘users.php’ and add the following code to it:
<?php
$mysqli = new mysqli("localhost", "db_user", "your_password", "your_db");
$action = $_GET["action"];
$result = "{'success':false}";
header('Access-Control-Allow-Origin: *');
header('Access-Control-Allow-Methods: GET, POST, OPTIONS');
header('Access-Control-Allow-Headers: Content-Type,x-prototype-version,x-requested-with');
echo($result);
?>
What we are doing here is first connecting to the database – you will have to replace these details with your own. Next we are grabbing the ‘action’ which will be passed into the API through the URL. For example: http://www.example.com/api/users.php?action=something. Eventually we will cycle through this ‘action’ variable to perform the appropriate action. When we want to log a user in we could make an Ajax request to ‘users.php?action=login’ or if we wanted to log a user out ‘users.php?action=logout’ and so on.
Before outputting the result, we are also setting our headers here. These headers are necessary to overwrite the default Cross Origin Resource Sharing options and to prevent the errors above. If you read the CORS article I linked above you will notice that you can also set these headers at the server level instead of directly in your PHP files.
At the end of this file we are outputting $result
. If you were to visit this page in your browser you would simply see {'success':false}
. This is a JSON formatted string that is telling us that whatever operation was just performed on the server was not successful. In later parts of this tutorial series we will of course be outputting different data through this JSON string that will indicate whether a user successfully logged in, what their session key is and so on. This same format can be used to send in large, complex data including any and all details we had stored about a user in the database.
This concludes the first part of this tutorial series. At this point we have our database set up and our API ready to be added to and interacted with. Stay tuned for the following posts in this series; here’s a link to Part 2!