If you’ve played any amount of games growing up you will have likely used a sprite in some form. Most peoples first hand experience with sprites would likely be through the web though. It’s not uncommon to create a ‘sprite sheet’ that looks something like this:
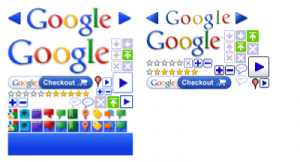
to hold different images for a website – usually small images like buttons and icons. The sprite sheet is one Google uses for their website (or has used at least).
The idea for the web is to cut down on HTTP requests: rather than hitting the server 10 times for 10 different images, only 1 request is sent. Of course, that one file will have a large file size (relatively speaking) but it will likely have a smaller file size than the sum of all the individual files. So smaller overall size + less HTTP requests = better performance. You can use a service like SpriteMe to easily create a sprite sheet for your website.
Once we have that big image loaded into memory, we then need to display only the section of the image we need for different buttons and icons. To do this we set the background-position property to correspond with the location of the image on the sprite sheet, for example:
#header {
background-image: url('images/myspritesheet.gif');
}
#header .button1 {
background-position: 0px 0px;
}
#header .button2 {
background-position: 0px -50px;
}
As I mentioned at the start, the concept of sprites is also heavily used in gaming to animate characters. Much more advanced techniques are used for more modern and complex games today (you’re certainly not going to see sprites used to animate character in Call of Duty), but for simple or retro games sprites are still used. A spritesheet for a game character might look something like this:

This is from a game I’m currently developing with Phaser for iOS and Android (it doesn’t usually have a big ugly line through it, that’s just a watermark). Each frame displays the character in a different stance, if we string these together and play them like a flip book it will look like the character is running, jumping or doing whatever we need.
It works exactly the way a projected film does, a bunch of still frames shown in quick succession will make a movie.
Using Sprites in Phaser
Using sprites to animate a character in Phaser works similarly to the way I described it is done in CSS above. Here’s what you need to do:
1. Make the spritesheet
First you will need to make your sprite sheet, just like the one I showed above. You will need to place the images side by side, making sure they all occupy the same space. In the example above each sprite occupies a space 38 pixels wide and 48 pixels high. It’s important that the width is maintained correctly, otherwise parts of your sprite will get cut off and parts of the next sprite will display in the wrong frame… kind of like this:
which won’t exactly give you the effect you’re after.
2. Load your spritesheet in the preload function
Before we can make use of the spritesheet, or any resources for that matter, we need to load them into the game within the preload function:
game.load.spritesheet('myguy', 'assets/myguy.png', 38, 48);
The parameters supplied for this function are:
- The key which will be used to refer to this spritesheet
- The path to the file
- The x width of each frame in your sprite
- The y width of each grame in your sprite
So if you had a spritesheet that was 200px x 30px and contained 4 frames (4 different character images) each 50px wide then you would instead use:
game.load.spritesheet('myguy', 'assets/myguy.png', 50, 30);
3. Add the spritesheet to the game
Once your spritesheet is loaded, you will need to add it to the game. To do this, add the following code within the create()function:
mysprite = this.game.add.sprite(15, 30, 'myguy');
mysprite.frame = 3;
This will add the sprite at the coordinates x = 15, y = 30 in your game. The sprite will be positioned such that only the 4th frame is showing, since we specified:
mysprite.frame = 3;
(counting starts at 0, not 1 so that’s why it is 3 and not 4). We can set this to be whatever we want (as long as there are enough frames of course). You would likely want to set this to be whatever your sprites idle or default stance is.
4. Create animations
The whole point of the sprite sheet is to make our character look like it is actually moving, so we need to create some animations somehow. To do this in Phaser is very simple, we just loop between different frames in the sprite by using the following code:
mysprite.animations.add('left', [0, 1, 2, 3], 10, true);
mysprite.animations.add('right', [5, 6, 7, 8], 10, true);
In the example above I am adding two different animations: left and right. For the left animation I supply the following array:
[0, 1, 2, 3];
this array corresponds to the frames I would like to play in the animation. These frames contain the poses for the character running in the left direction. So what we’re saying here is to loop through frames 0, 1, 2 and 3 at 10 frames a second (because we supplied 10 as a parameter) while this animation is playing.
Similarly for the right animation we are looping through frames 5, 6, 7 and 8.
5. Play the animations
Finally, the only thing left to do is actually play the animation. To trigger the animation to start looping we just need to call:
mysprite.animations.play('left');
or
mysprite.animations.play(‘right’);
and our animation will start looping. When you want the animation to stop playing you simply call:
mysprite.animations.stop();
and you may also want to reset the frame to whatever your default state is:
mysprite.frame = 4;
and that’s it!
In this example I’ve talked about creating simple running animations but you can do a lot more as well. Combine it with physics in Phaser and you can create great looking jumping animations, or even diving, sliding, punching and slicing animations. All you need is the right sprite sheet. If you can’t create these sprites on your own, Graphic River have a bunch available for very affordable prices.