If you come from an Ionic 1.x or AngularJS background, then you would be used to handling navigation through routing with URLs, states, and so on. In the newer versions of Ionic, it will actually be possible to use this style of navigation with Ionic, but we will be focusing on the “standard” navigation when Ionic is used with Angular in this tutorial.
The focus in Ionic/Angular applications is using a navigation stack, which involves pushing views onto the navigation stack and popping them off. Before we get into the specifics of how to implement this style of navigation in Ionic, let’s try to get a conceptual understanding of how it works first.
Pushing and Popping
Imagine your root page is a piece of paper that has a picture of a cat on it, and you put that piece of paper on a table. It is the only piece of paper currently on the table and you are looking down on it from above. Since it is the only piece of paper on the table right now, of course you can see the picture of the cat:
Drawn on my laptops trackpad, no judging
Now let’s say you want to look at a different piece of paper (i.e. go to a different page), to do that you can push it onto the stack of papers you have. Let’s say this one is a picture of a dog, you take that piece of paper and place it over the top of the picture of the cat:
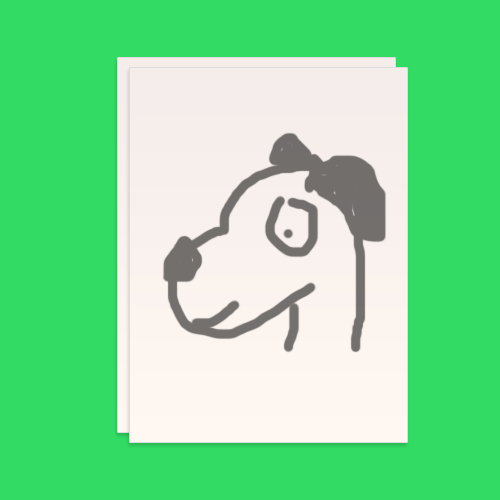
The cat is still there, but we can’t see it anymore because it is behind the dog. Let’s take it even further and say that now you want to push another piece of paper, a cow, it would now look like this:
This one looks more like a Bellsprout than a cow
Both the cat and the dog are still there, but the cow is on top so that is what we see. Now let’s reverse things a bit. Since all of the pieces of paper are stacked in the order they were added we can easily cycle back through them by popping. If you want to go back to the picture of the dog you can pop the stack of papers, removing the piece of paper that is currently on top (the cow). If you want to go back to the picture of the cat you can pop the stack of papers once more to remove the piece of paper that is now on top (the dog). Now we’re back to where we started.
I’m sure you can see how this style of navigation is convenient for maintaining navigation history and it makes a lot of sense when navigating to child views, but it doesn’t always make sense to push or pop. Sometimes you will want to go to another page without the ability to go directly back to the page that triggered the change (a login screen that leads to the main app for example, or even just different sections of an app available through a menu).
In this case, we could change the root page which, given our pieces of paper on the table analogy, is like disregarding the other stack of papers we have and just focusing on a new piece of paper on the table:
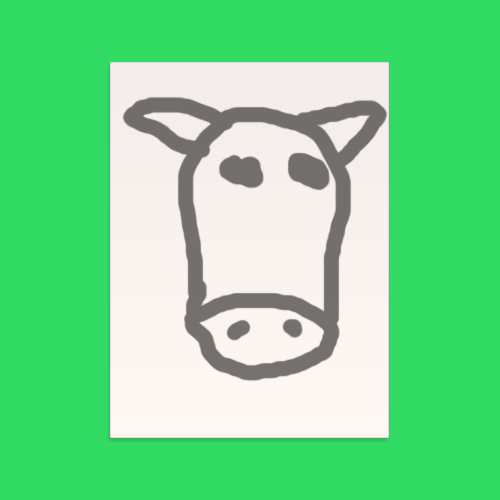
In the example above, I’ve set the cow page as the root page, so rather than being on top of the other pages, it’s all by itself.
When should you push and when should you set the root page?
At first, it may be hard to understand whether you should set the root page to navigate to a different page or push the view. In general, if the view you want to switch to is a child of the current view, or if you want the ability to navigate back to the previous view from the new view, you should push. For example, if I was viewing a list of artists and tapped on one I would want to push the details page for that artist. If I was going through a multi-page form and clicked ‘Next’ to go to page 2 of the form, I would want to push that second page.
If the view you are switching to is not a child of the current view, or it is a different section of the application, then you should instead change the root page. For example, if you have a login screen that leads to the main application you should change the root page to be your main logged in view once the user has successfully authenticated. If you have a side menu with the options Dashboard, Shop, About and Contact you should set the root page to whichever of these the user selects.
Keep in mind that the root page is different to the root component, typically the root component (which is defined in app.component.ts) will declare what the root page is – the root page can be changed throughout the application, the root component can not.
Basic Navigation in Ionic
Ok, so now we’re going to get into a more practical Ionic example and look at how to push, pop, set the root page and even how to pass data between pages. It’s all pretty simple really.
An important part of all this is the NavController. You will often see this imported by default in your Ionic 2 applications:
import { NavController } from 'ionic-angular';
and it also needs to be injected into the constructor:
@Component({
templateUrl: 'home.html',
})
export class MyPage {
constructor(private navCtrl: NavController) {
}
}
A reference to the NavController is created so that we can use it anywhere in the class. So let’s take a look at how to push and pop.
To push a page, that means to make it the current page, you can do something like this:
this.navCtrl.push(SecondPage);
This uses the reference to the NavController we created before, and all you need to supply to it is a reference to the component that you want to navigate to, which you will need to make sure you also import at the top of the file:
import { SecondPage } from '../second-page/second-page';
and that’s it, your app should switch to the new page whenever the push code is triggered. Keep in mind that if you are using lazy loading with @IonicPage
you should instead push pages like this:
this.navCtrl.push('SecondPage');
and there is no need to import the page. If you don’t know what @IonicPage
is yet, don’t worry about that for now, just use the first navigation method I mentioned.
When you push a page, a ‘Back’ button will automatically be added to the nav bar, so you often don’t need to worry about using pop to navigate back to the previous page since the ‘Back’ button does this automatically for you.
There may be circumstances where you do want to manually pop a page off of the navigation stack though, in which case you can use this:
this.navCtrl.pop();
Easy enough right? As I mentioned before there is still another way to change the page and that is by setting the root page. If you take a look at your app.ts file you will notice the following line:
rootPage: any = MyPage;
Declaring a member variable rootPage in the root component will set the root page. To change the root page at any point throughout the application, you can use our mate the NavController – all you have to do is call the setRoot function like this:
this.navCtrl.setRoot(SecondPage);
Passing Data Between Pages in Ionic
A common requirement of mobile applications is to be able to pass data between pages. In Ionic/Angular, this can be done using NavParams. First, you must pass through the data you want within the push call (this can also be done when using setRoot):
this.navCtrl.push(SecondPage, {
thing1: data1,
thing2: data2,
});
This is exactly the same as what we were doing before, except now there is an extra parameter which is an object that contains the data we want to send through to SecondPage. Then on the receiving page, we need to import NavParams and inject it into our constructor:
import { Component } from '@angular/core';
import { NavController, NavParams } from 'ionic-angular';
@Component({
templateUrl: 'second-page.html'
})
export class SecondPage {
constructor(private navCtrl: NavController, private navParams: NavParams){
}
}
Then you can grab the data that was passed through by doing the following:
this.navParams.get('thing1');
Summary
It might be a little difficult to get your head around at first, especially if you’re not familiar with the pushing and popping concept, but once you get used to it I think this is a very simple and powerful form of navigation.