One thing I really like about Ionic is that the default components look great right out of the box. Everything is really neat, sleek and clean…
…but also maybe a little boring. I like simple, simple is great – but you probably don’t want your app to look like every other app out there. Here’s an example of a couple Ionic 2 applications that I’ve done some custom styling for:
Giflist is currently available on app stores, just click the image
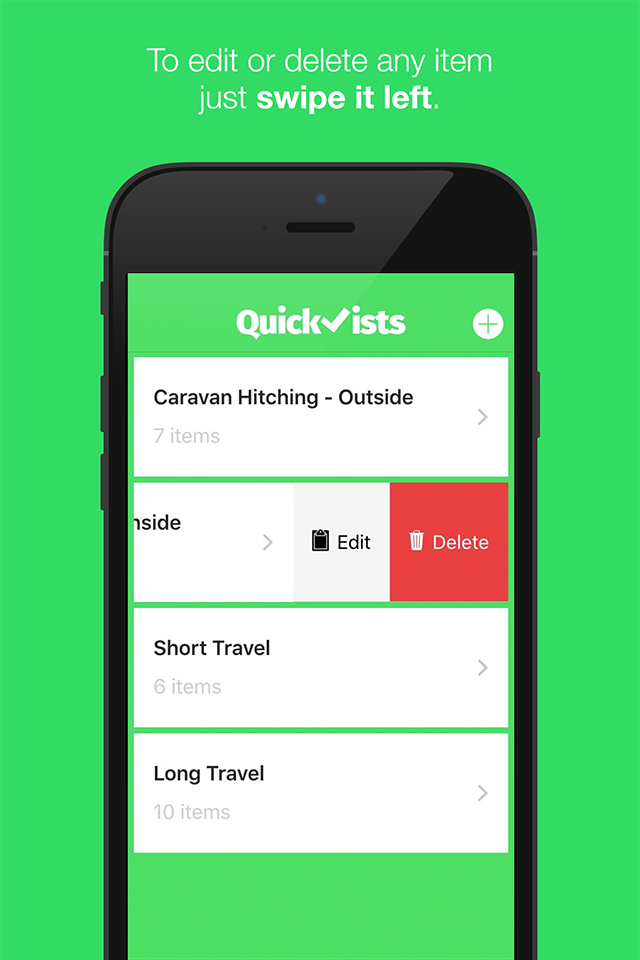
I certainly don’t claim to be some design guru, but I think the themes I’ve created for these applications are visually pleasing and help give the apps some character.
So, in this tutorial I’m going to show you the different ways you can customise your Ionic 2 applications, and the theory behind theming in general.
Introduction to Theming in Ionic 2
When styling an Ionic 2 application, there is nothing inherently different or special about it – it’s no different than the way you would style a normal website. I often see questions like “Can I create [Insert UI element / interface] in Ionic?” and the answer is generally yes. Could you do it on a normal webpage? If you can then you can do it in Ionic as well.
A lot of people may be used to just editing CSS files to change styles, but there is some added complexity with Ionic, which is primarily due to the fact that it uses SASS. Again, SASS isn’t specific to Ionic or mobile web app development – it can also be used on any normal website – but many people may not be as familiar with SASS as they are with CSS.
If you’re not already familiar, .scss is the file type for SASS or Syntactically Awesome Style Sheets. If this is new to you, you should read more about what SASS is and what it does here. For those of you short on time, what you put in your .scss files is exactly the same as what you would put in .css files, you can just do a bunch of extra cool stuff as well like define variables that can be reused in multiple areas. These .scss files are then compiled into normal .css files (it’s basically the same concept we use in Ionic 2, where we code using all the fancy new ES6 features, but that is then transpiled into ES5 which is actually supported by browsers now.
When theming your application, you’re mainly going to be editing your .html templates and .scss stylesheets – you will NEVER edit any .css files directly. The .css files are generated from the .scss files, so if you make any changes to the .css file it’s just going to get overwritten.
If you take a look at the files generated when you create a new Ionic 2 project, you will see some .scss files so, let’s quickly run through what their purpose is.
- app.scss is the main .scss file. It is used to declare any styles that will be used globally throughout the application. Although it is the “main” .scss file, you won’t likely use it often – most of the styling will happen in the component specific .scss files.
- variables.scss is used to modify the apps shared variables. Here you can edit the default values for things like the $colors map which sets up the default colours for the application, $list-background-color, $checkbox-ios-background-color-on and so on. For a list of all the variables that you can overwrite, take a look at this page
On top of these .scss files, you will also have one for each component you create (or at least you should). To refresh your memory, most components you create in Ionic 2 will look like this:
- mycomponent
- mycomponent.ts
- mycomponent.html
- mycomponent.scss
We have a class definition in the .ts file, the template in the .html file and any styles for the component in the .scss file. Although it’s not strictly required, you should always create the .scss file for any components that have styling, rather than just defining the style in the app.scss file.
NOTE: By using the ionic g page MyPage
command all the files you need will automatically be set up for you.
Why shouldn’t you put styles in app.scss? Well you could just put all of your styles in the app.scss file and everything would work exactly the same, but there are two major benefits to splitting your styles up in the way I described above:
- Organisation – Splitting your code up in this way will keep the size of your files down, making it a lot easier to maintain. Since all of the styles for a particular component can be found in that components .scss file, you’ll never have to search around much.
- Modularity – one of the main reasons for the move to this component style architecture in Angular 2 and Ionic 2 is modularity. Before, code would be very intertwined and hard to separate and reuse. Now, almost all the code required for a particular feature is contained within its own folder, and it could easily be reused and dropped into other projects.
Since each component can be given its own selector, i.e:
@Component({
selector: 'my-page',
templateUrl: 'my-page.html'
})
You can easily use SASS nesting to make sure rules you create only affect that one component, i.e:
my-page {
img {
width: 100%:
height: auto;
}
}
With the above .scss file, only images in the MyPage component will be affected by that rule. You can also nest within the ios
and md
classes to specifically only target iOS or Android, i.e:
.ios, .md {
my-page {
img {
width: 100%:
height: auto;
}
}
}
This will apply the styles to both iOS and Android, but you can target each class specifically if you like. Now that we’ve gone over the theory, let’s look at how to actually start styling our Ionic 2 applications.
Methods for Theming an Ionic 2 Application
I’m going to cover a few different ways you can alter the styles in your application. It may seem a little unclear what way to do things because in a lot of cases you could achieve the same thing using any of the following 3 methods I’m about to list. In general, you should try to style your applications using the methods below in the order they appear. If you can do it using method #1 use that, if not use method #2 and finally use method #3 if you need to.
1. Attributes
One of the easiest ways to change the style of your application is to simply add an attribute to the element you’re using. As I mentioned above, SASS is used to define some colours, and these are:
- primary
- secondary
- danger
- light
- dark
Ionic provides some defaults for what these colours are, but you can also override each of these to be any colours you want (which we will talk about soon). So if you add the primary attribute to most elements it will turn blue, or if you add the danger attribute it will be a red colour.
So for example, if I wanted to use the secondary colour on a button I could do this:
<button color="secondary"></button>
or if I wanted to use the secondary colour on a the nav bar I could do this:
<ion-navbar color="secondary"></ion-navbar>
Keep in mind that these attributes aren’t limited to just changing the colour of elements, some attributes will also change things like the position:
<ion-navbar color="secondary">
<ion-buttons end>
<button ion-button color="primary">
I'm a primary coloured button in the end position of the nav bar
</button>
</ion-buttons>
</ion-navbar>
whether or not a list should have borders:
<ion-list no-lines></ion-list>
or even whether a list item should display an arrow to indicate that it can be tapped:
<ion-item detail-none></ion-item>
There’s a bunch more of these attributes, so make sure to poke around the documentation when you are using Ionics built-in components. The no-lines attribute is a real easy way to remove lines from a list, but if you didn’t know this attribute existed (which is quite possible) then you’d likely end up creating your own custom styles unnecessarily.
2. SASS Variables
The next method you can use to control the style of your application is by changing the default SASS variables. These are really handy because it allows you to make app wide style changes to specific things. I touched on SASS variables before, but basically in your .scss files you can do something like this:
$my-variable: red;
and then you could reference $my-variable anywhere in the .scss file. So for example if you wanted to make the background colour on 20 different elements red, rather than doing:
background-color: red;
for all of them, you could instead do this:
background-color: $my-variable;
The benefit of this is that now if you wanted to change the background color from red to green, all you have to do is edit that one variable – not every single class you have created.
Ionic defines and uses a bunch of these variables, and you can easily overwrite them to be something else. Let’s take a look at a few:
- $background-color
- $link-color
- $list-background-color
- $list-border-color
- $menu-width
- $segment-button-ios-activated-transition
You can look at the documentation for more information on these and what they default to, but it’s pretty clear by their name what they do. As you can see by the last example there, they even get very specific.
Editing these variables is really simple, just open variables.scss and insert your own definitions. Here’s an example of one of my variables.scss files:
$colors: (
primary: #387ef5,
secondary: #32db64,
danger: #f53d3d,
light: #f4f4f4,
dark: #222
);
$list-background-color: #fff;
$list-ios-activated-background-color: #3aff74;
$list-md-activated-background-color: #3aff74;
$checkbox-ios-background-color-on: #32db64;
$checkbox-ios-icon-border-color-on: #fff;
$checkbox-md-icon-background-color-on: #32db64;
$checkbox-md-icon-background-color-off: #fff;
$checkbox-md-icon-border-color-off: #cecece;
$checkbox-md-icon-border-color-on: #32db64;
Notice the use of md here, this stand for material design and is used for Android. Ionic 2 seamlessly adapts to the conventions of the platform it is running on with little to no style changes required from you – for Android this means material design is used.
The great thing about editing these default SASS variables is that you can, with one change, make all the changes necessary everywhere in the app. Some variables use the values of other variables, so if you wanted to just do this manually with CSS you would probably need to make a lot of edits to get the effect you wanted.
3. Custom Styles
Before we talked about using attributes to change the colours of elements. Given that you can override these attributes to whatever you like, it’s a good approach to set the primary, secondary, danger etc. variables to match the colour palette of your design, and then use those to set the styles of elements, rather than defining custom CSS classes.
But, sometimes there will come a time where you need to define some plain old CSS classes to achieve what you want. You can either define these custom classes in app.scss if the class will be used throughout the application, or in an individual component’s .scss file if it is only going to be used for one component.
Of course, you can also define custom styles on the element directly by using the style tag, but make sure you use this sparingly.
Summary
As you can see, there are a few different ways you can change the styling of your Ionic 2 applications. In general, it’s best to do as little as possible to achieve what you need. Try to achieve as much as you can with attributes and SASS variables, because not only will this make your life easier, it will also make Ionic’s job easier (which in turn, is also good for you!).
As I mentioned before, Ionic seamlessly adapts to the UI conventions of both iOS and Android, so the more “hacky” or “brute force” your solution for styling is, the greater chance you have of breaking this behaviour.